Lecture 1
Course overview & Python review
Your professor:
- Graduated from UMBC,majoring in Information Systems, minor in Computer Science
- Master's degree from UMBC in Cybersecurity
- Graduate certificate in Data Science
- I work at a large consulting company as a software engineer, have worked at a start-up
- Outside of work, I enjoy working on open source software, and traveling.
Now it's your turn:
- Your name
- Year
- Major (and minor if applicable)
- What do you want to learn or get out of this class?
- One thing that you like and/or like to do
Course basics
- For all majors other than CS/CE and pre-CS/CE
- Prerequisites:
- CMSC 201 (with a C or better)
- Is not equivalent to CMSC 202, Computer Science II
- Does not count as a CMSC elective for CS majors
- 3 credits, no lab
- Second class in the new Computing Minor (designed for non-computer science, non-computer engineering majors interested in pursuing further study in applied computing).
- Otherwise, this class is worth nothing outside of the 3 credits. Oh and, the educational value of course.
- Our TA is TBD.
- Course website: https://rjzak.github.io/cmsc-210/
- We'll use Blackboard for a few things here and there.
- Office hours are by appointment, or after class
When do I use...
UMBC gl system? |
Blackboard? |
My own computer + Github? |
Never |
Announcements |
When you submit an assignment. |
Grading scheme
There are seven assignments, mostly programming and they are due approximately every other week.
Type | Points Each | Subtotal |
Assignment 1 | 100 | 100 |
Assignments 2 - 7 | 150 | 900 |
Total | 1,000 |
Final exam
There isn't one. But we may meet for some activity, TBD.
Submissions & lateness policy
- Assignments will be due on Wednesday before midnight, unless otherwise announced.
- Late assignments will receive a zero.
Academic integrity
- Never submit work done by someone else as your own.
- If it's another student's work, you will both get a zero on the assignment, at minimum.
- I must report integrity violations to UMBC’s Academic Misconduct Reporting Database.
- Read the UMBC Undergraduate Student Academic Conduct Policy
There are ways to get help if you're struggling!
- You can talk to your classmates about concepts
- You can talk to your TA or come to my office hours
- You can compare the output of your program to someone else's ("Did I do this right?")
- You can work on practice problems together.
- You can get help if your computer is having problems at the Technology Support Center
Assignment Zero
Get familiar with Blackboard and do some basic admin there...
- Fill-out the First Day Survey
- Check Blackboard announcements
- Join the Discord server (if you want)
- Create an account on Github
- Should be quick
Course objectives
1. Become a better software engineer
- Learn Python: more and better
- Develop and structure more complex programs
- Understand software engineering fundamentals:
- tooling
- design
- distribution
- debugging
- testing
- version control
Course objectives
2. Build useful things
- Data: acquisition, exploration, & visualization
- HTML and CSS
- Web scraping
- Web application development
- Machine learning
Why?
The skills you learn here can help you in future courses, in research and internships, and in your career.
What questions do you have?
Python is an interpreted, dynamically-typed, and strongly-typed programming language with garbage collection and significant whitespace.
Interpreted 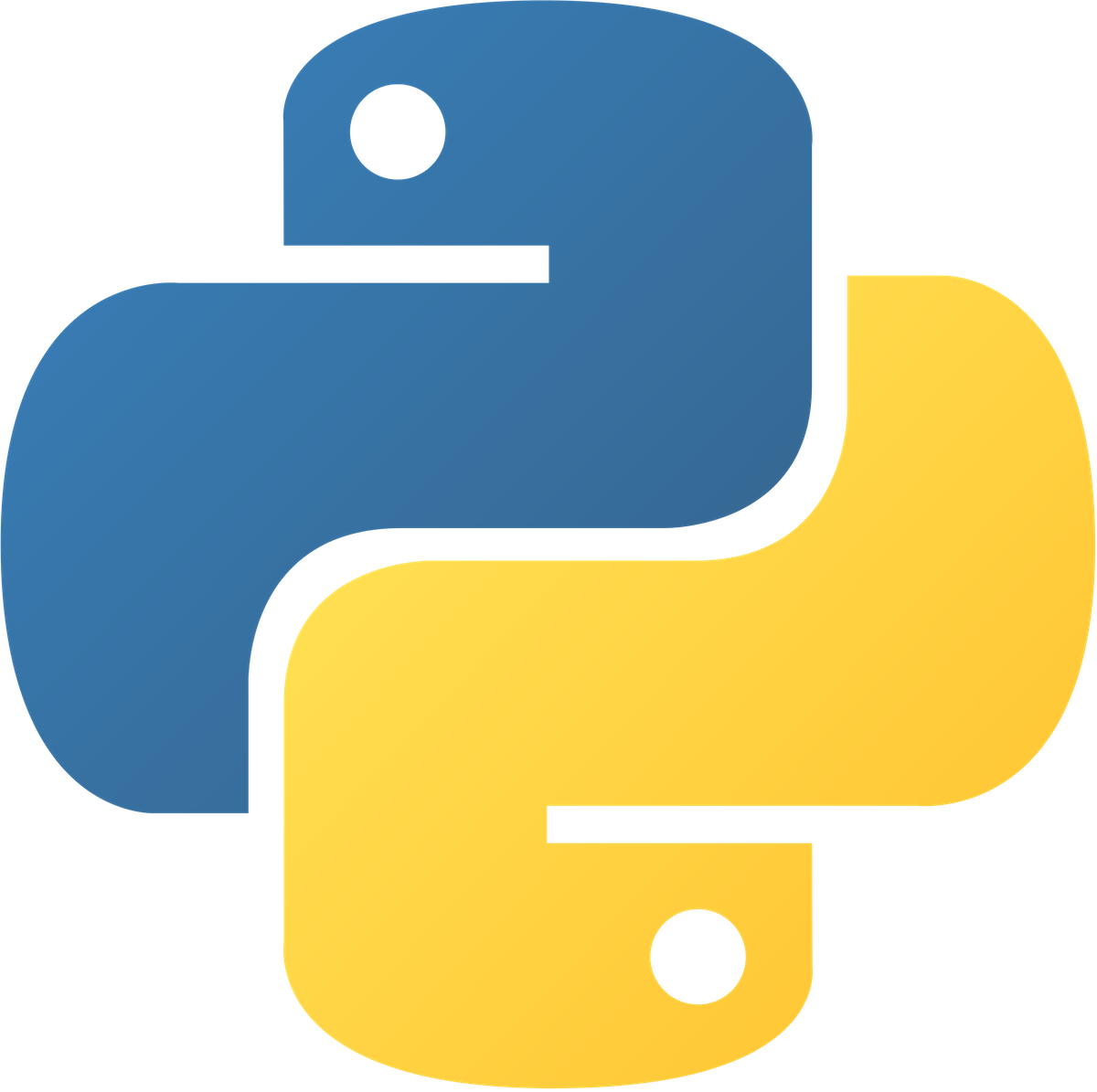
- Program ☞ Source Code
- To run the program, you require an interpreter
- Examples: Python, JavaScript
Compiled
- Program ☞ Executable
- No interpreter needed to run the program.
- Examples: C++, Java
Dynamic Types 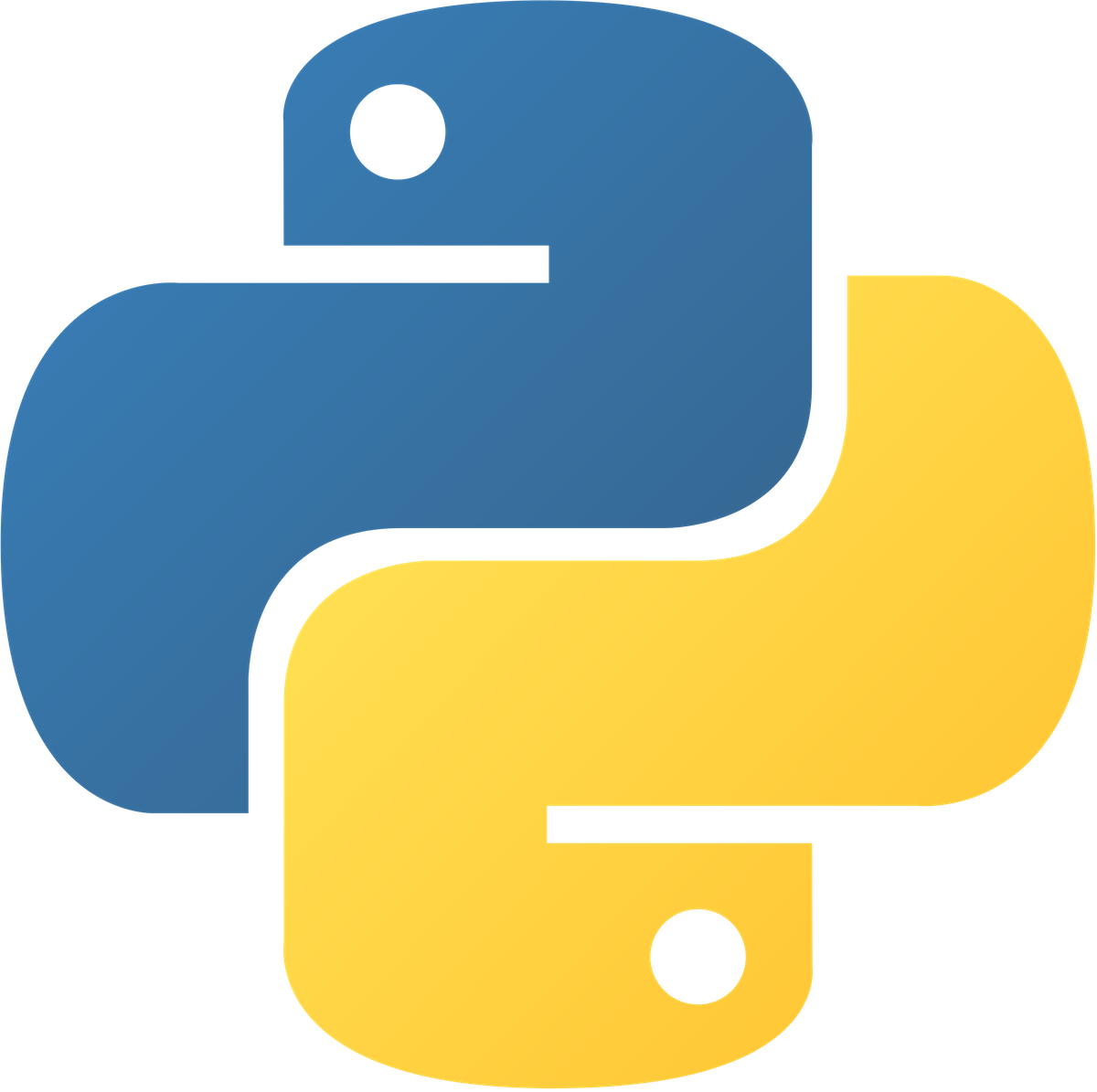
- Types are checked at runtime.
Static Types
- Types checked at compile time (or before running).
Strong Types 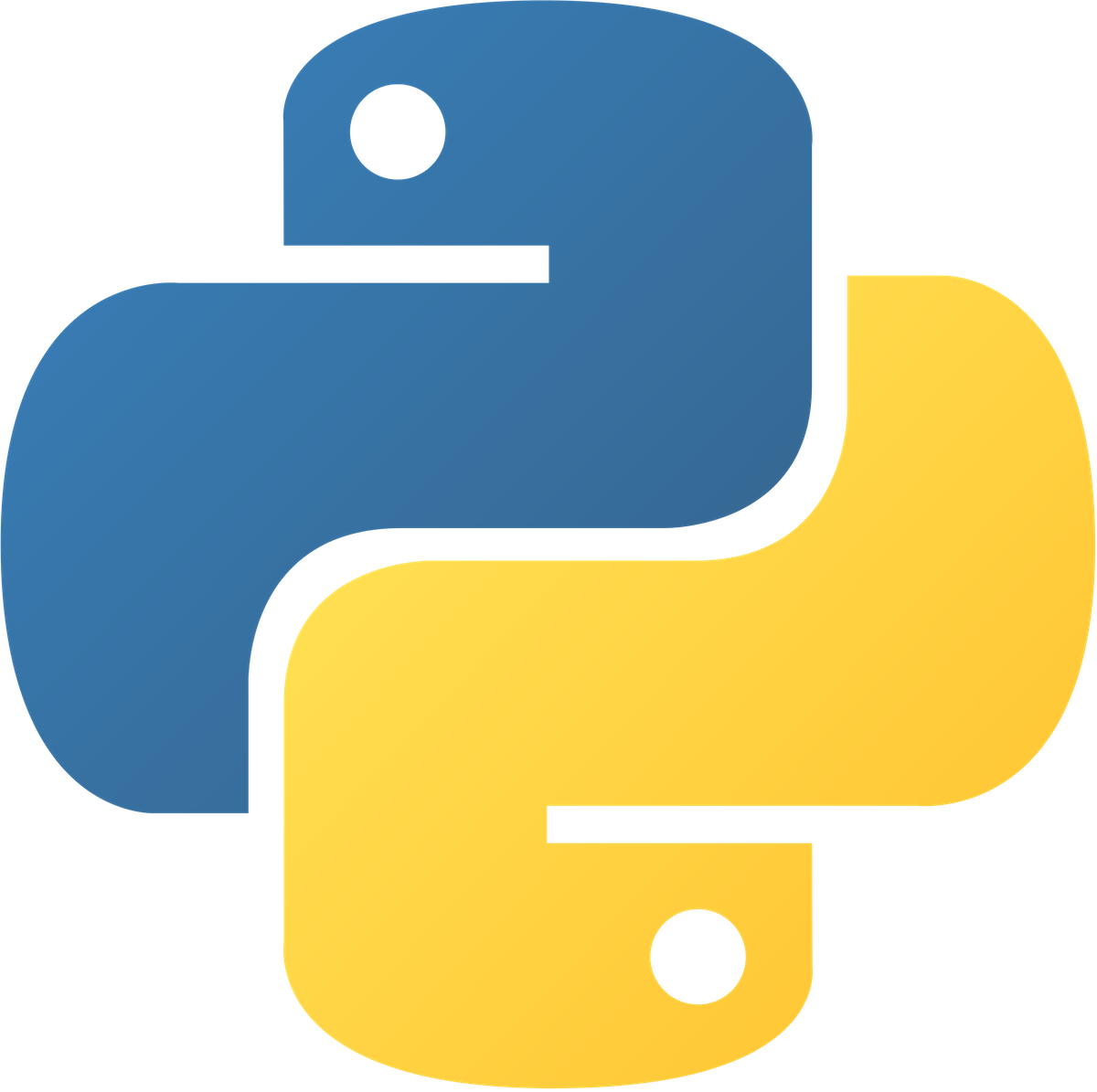
- Compiler or interpreter will not try to correct type errors by coercing types.
>>> 1 + "1"
TypeError
Weak Types
- Compiler or interpreter may implicitly coerce types to avoid type errors.
>>> 1 + "1"
"11"
Automatic memory management 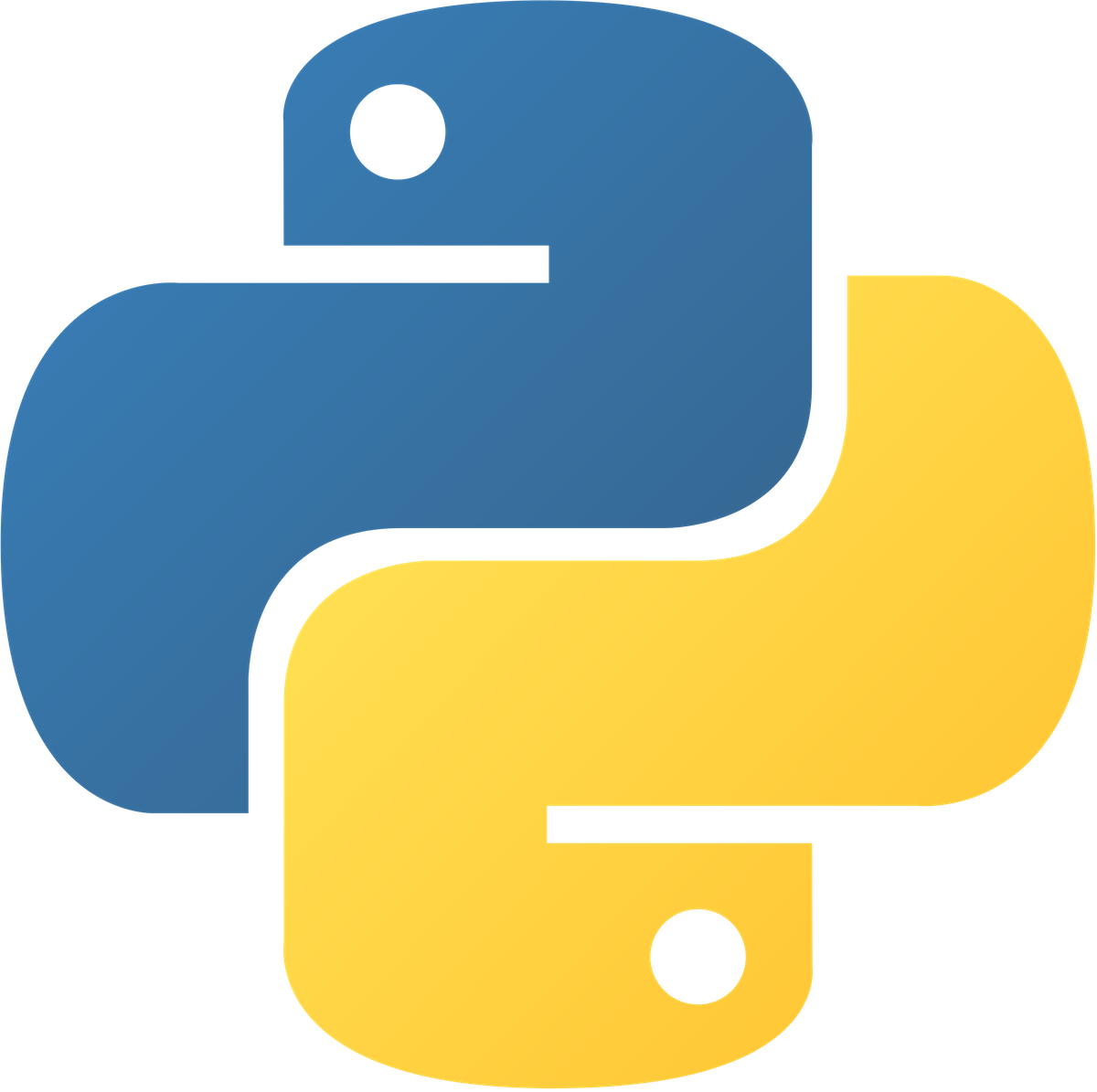
- Memory is managed for you by the Python runtime.
- The garbage collector reclaims memory when no longer needed.
Manual memory management
- It's up to you to manage you own memory.
- The programmer is responsible for allocating and de-allocating memory.
Blocks expressed by indentation 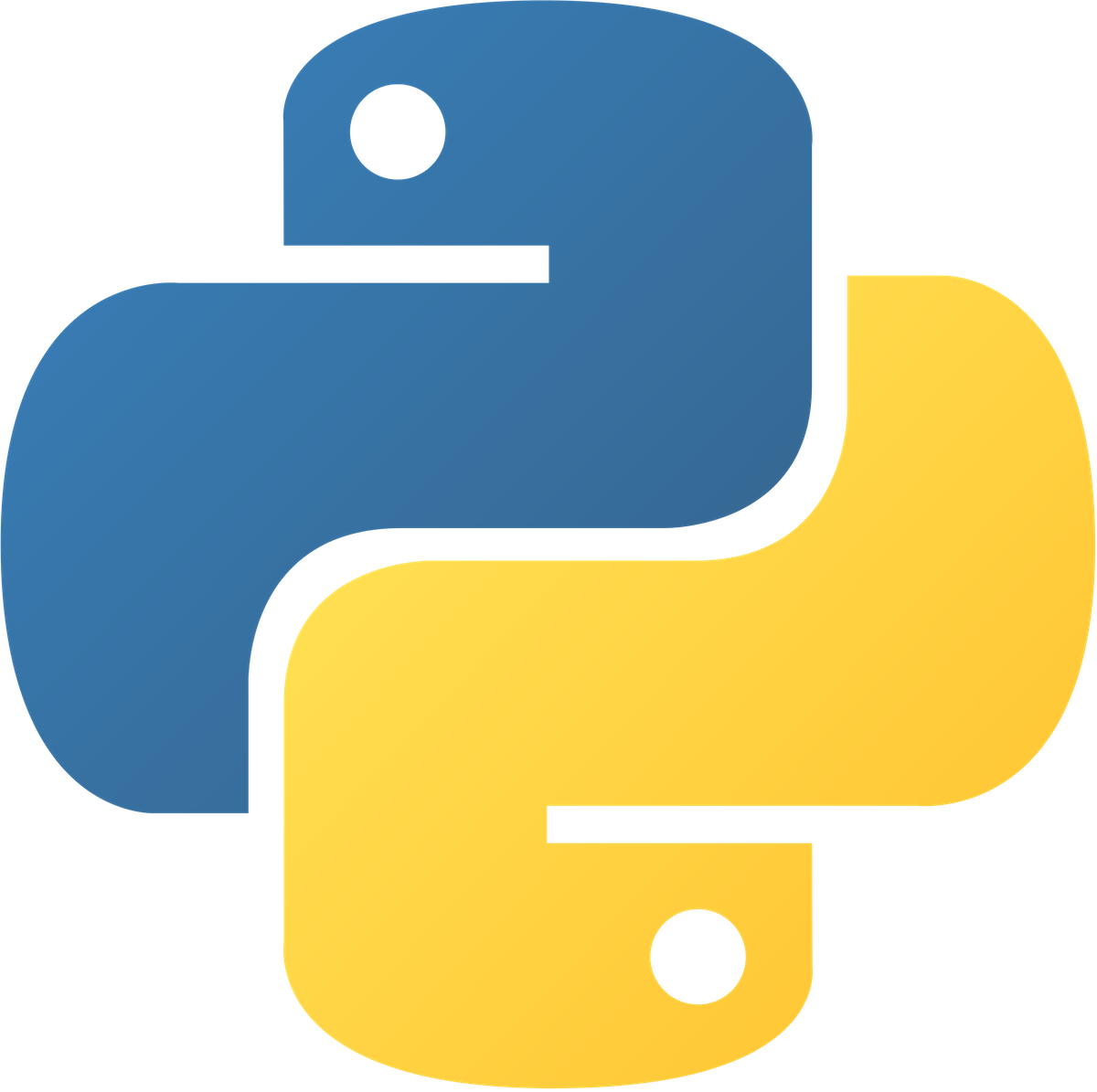
if some_number == 2:
print("It's two!")
print("All done.")
Blocks are surrounded by characters
if (some_number == 2) {
print("It's two!")
}
print("All done.")
Numeric Types
# Integer (whole number)
a = 255
# Float (decimal type)
b = 255.1
c = float(255) # Or c = 255.0
Numeric Operations
- Addition (
+
)
- Subtraction (
-
)
- Multiplication (
*
)
- Division (
/
, this always gives you a float)
- "Floor" division (
//
, this always gives you an int)
- Modulo (
%
, gives you the remainder)
- Rounding (
round(1 / 3, 2)
)
String Type
a = "I am a string"
b = 'I am a string'
c = """I am a string""" # a, b, and c are equal.
# Backslashes used for escaping:
d = "I am a string \"with a quote\"."
e = """I am a
multiline string."""
String Operations
- Concatenation
"left " + "right"
- Changing case
"a string".upper().lower()
- Stripping
" a string ".strip()
- Splitting
"A, B, C".split(", ")
- Joining
", ".join(["A", "B", "C"])
Control structures: decision
# Simple:
if a == 2:
print("A is 2.")
# Complex:
if a == 2:
print("A is 2.")
elif a == 3:
print("A is 3.")
else:
print("A is not 2 or 3.")
Control structures: iteration
# WHILE: keep looping until some condition is met.
a = 1
while a < 4:
a = a + 1
# FOR: go through each item in an iterable.
b = 0
for number in [1, 2, 3]:
b = b + number
Iterables: lists
- Compound type
- Mutable:
>>> my_list = [1, 2, 3]
>>> my_list.append(4)
>>> my_list
[1, 2, 3, 4]
- Ordered
- Random access to any element or subset
>>> my_list = [1, 2, 3, 4]
>>> my_list[0]
1
>>> my_list[1]
2
>>> my_list[-1]
4
>>> my_list[0:2]
[1, 2]
Iterables: more lists
- Lists have a length
>>> my_list = [1, 2, 3]
>>> len(my_list)
>>> 3
- Can test for membership:
>>> my_list = [1, 2, 3, 4]
>>> 4 in my_list
True
>>> 5 in my_list
False
Dictionaries
- Compound type
- Unordered set of key-value pairs
- Keys must be unique
- Keys must be of an immutable and hashable type
- Values do not have to be unique
- Values may be immutable or mutable
pals = {
"Bob": 2,
"Carol": 1,
"Kim": 0
}
# How many times have I hung out with Bob?
pals["Bob"] # I know Bob is in there
pals.get("Bob") # I don't know
pals.get("Bob", 0) # I want a default number.
# Bob and I are no longer pals :-(
del pals["Bob"]
# I want a list of my friends' names
pals.keys()
# How many times have I hung out with all friends?
sum(pals.values())
# Am I pals with Bob?
"Bob" in pals
If you still feel shaky...